CSS Grid Tutorial
Contents of CSS Grid Tutorial
The mobile internet is a major challenge for website developers. Mobile devices such as tablets and smartphones have different screen resolutions than desktop computers and laptops. These different displays and screen resolutions need to be considered when designing web pages.
Responsive Web Design ensures that a website looks great and is usable on any device at any screen resolution. Responsive layouts automatically adjust to the available screen resp. window size.
The CSS Grid is a grid of imaginary lines. It is used to divide the layout of a web page into rows and columns. Grid cells are the intersections of rows and columns. A grid cell is bounded by four grid lines and is the smallest unit in the grid container. This layout grid can be used to effectively design complex, nested layouts.
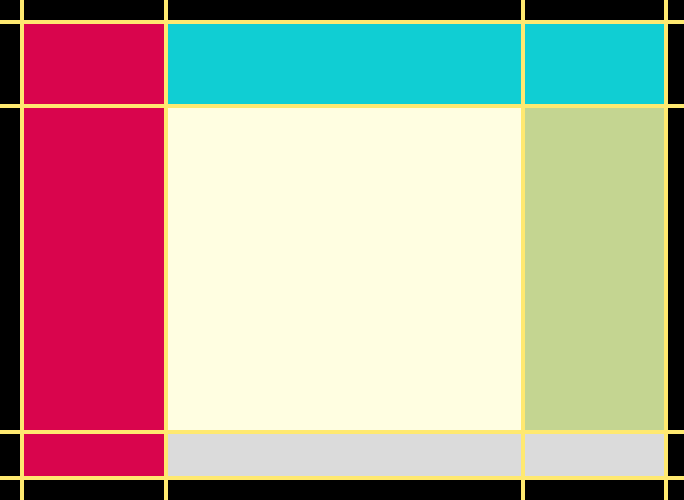
Basic Function of CSS Grid
CSS Grid works with a parent element (grid container) in which the grid is defined. The grid-template-columns and grid-template-rows properties create the grid and draw the imaginary grid lines.
If you want to create spaces between the columns or rows of the grid, you can set the width of the grid lines using the gap property of the grid container.
You can specify one or two values for gap.
-
one value
The value defines the size of space between the columns and rows. -
two values
The first value specifies the vertical space between the rows (row-gap), the second value specifies the horizontal space between the columns (column-gap).
The gap property is a shorthand for the column-gap and row-gap properties (formerly grid-gap, grid-column-gap and grid-row-gap).
Note:The spacing is only created between the columns or rows. The first and last grid lines in horizontal and vertical directions remain unchanged.
When child elements are inserted into the grid container, the content is automatically inserted into the grid. By default, elements in the grid container are aligned row by row from top left to bottom right.
The following example creates a CSS grid with three rows and four columns. The first row has a height of 12em, and the third row has a height of 6em. The middle row occupies the remaining space.
Example CSS/* Define the Grid Container */
.grid-container
{
display
: grid
;
grid-template-rows
: 12em 1fr 6em
;
grid-template-columns
: auto auto auto auto
;
gap
: 1.0em
;
padding
: 0
;
background
: #dbdbdb
;
}
.grid-container > div
{
font-size
: 1.25em
;
text-align
: center
;
background
: #94bbcc
;
color
: #000000
;
border
: 1px
solid
#000000
;
}
<div class=
"grid-container"
>
<div>Box 1
</div>
<div>Box 2
</div>
<div>Box 3
</div>
<div>Box 4
</div>
<div>Box 5
</div>
<div>Box 6
</div>
<div>Box 7
</div>
<div>Box 8
</div>
<div>Box 9
</div>
<div>Box 10
</div>
<div>Box 11
</div>
<div>Box 12
</div>
</div>
This background represents the screen and is not part of the sample code.
To make the effect more visible, the output window has a height of 40em. The grid container has a height of 100% of the output window.
Position Items in the Grid
CSS Grid also allows you to position items anywhere in the grid. To achieve this, the CSS properties
- grid-row-start and grid-row-end resp.
- grid-column-start and grid-column-end
are used to tell the child elements where they should be placed in the grid. For these CSS properties, there are also the shorthand notations
- grid-row resp.
- grid-column
The start and end line of the grid are separated by a slash /.
Note:Positions are made using grid lines, not grid columns or grid rows.
The Example Layout shown above has four columns and three rows. It therefore has five vertical and four horizontal grid lines. If an element is to extend across the entire width, then it spans from grid line 1 (0%) to 5 (100%).
3 Column Layout with Header and Footer
The following code example shows how to position the elements in the grid to create a 3-column layout with header and footer.
/* 3 Column Layout using CSS Grid */
.gridBox
{
display
: grid
;
grid-template-rows
: 4em
1fr
2em
;
grid-template-columns
: 15%
auto
25%
;
gap
: 0
;
padding
: 0
;
background
: transparent
;
}
.gridBox > nav
{
grid-column-start
: 1
;
grid-column-end
: 2
;
grid-row-start
: 1
;
grid-row-end
: 4
;
padding
: 0 0.75em
;
font-size
: 1.25em
;
text-align
: center
;
background
: #600d0d
;
color
: #000000
;
}
.gridBox > header
{
grid-column-start
: 2
;
grid-column-end
: 4
;
grid-row-start
: 1
;
grid-row-end
: 2
;
padding
: 0 0.75em
;
font-size
: 1.25em
;
text-align
: center
;
background
: #ffffd2
;
color
: #000000
;
}
.gridBox > main
{
grid-column-start
: 2
;
grid-column-end
: 3
;
grid-row-start
: 2
;
grid-row-end
: 3
;
padding
: 0 0.75em
;
font-size
: 1.25em
;
text-align
: center
;
background
: #94bbcc
;
color
: #000000
;
}
.gridBox > aside
{
grid-column-start
: 3
;
grid-column-end
: 4
;
grid-row-start
: 2
;
grid-row-end
: 3
;
padding
: 0 0.75em
;
font-size
: 1.25em
;
text-align
: center
;
background
: #e5f3d8
;
color
: #000000
;
}
.gridBox > footer
{
grid-column-start
: 2
;
grid-column-end
: 4
;
grid-row-start
: 3
;
grid-row-end
: 4
;
padding
: 0 0.75em
;
font-size
: 1.25em
;
text-align
: center
;
background
: #dfdfdf
;
color
: #000000
;
}
<div class=
"gridBox"
>
<header>Headline
</header>
<main>Main Content of the Page
</main>
<aside>Info Box
</aside>
<nav>Navigation of the Page
</nav>
<footer>Footer
</footer>
</div>
This background represents the screen and is not part of the sample code.
To make the effect more visible, the output window has a height of 40em. The grid container has a height of 100% of the output window.
Recommendation
In this example, the width for the navigation and the right sidebar (Info Box) is specified as a percentage. In practice, it is usually better to use a relative unit such as em.
In the sample HTML code, the main content comes before the navigation. The info box and the footer are placed at the bottom of the document. This improves accessibility and SEO (Search Engine Optimization).